Use This One Trick to Find SuperMemo's Element Location
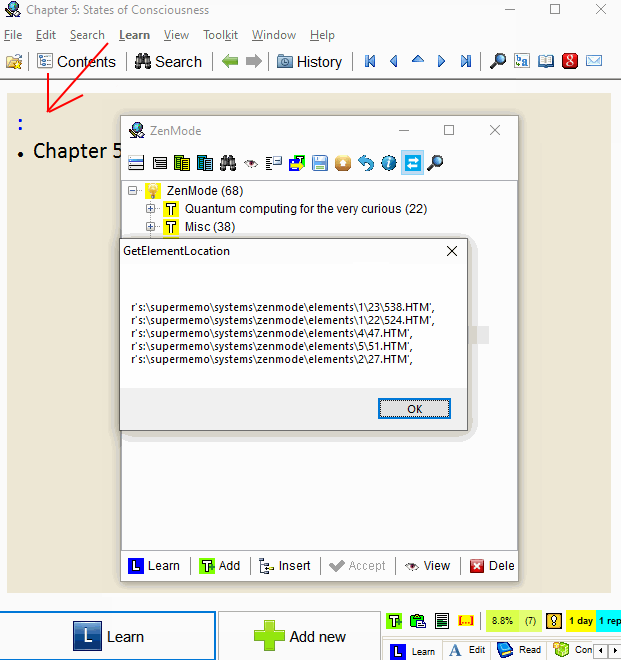
ctrl+c
to copy the meta-data of the current Element.Introduction
One day I found that with ctrl+c
, I could copy the current Element’s meta-data:
|
|
The most important field of our interest is HTMFile=s:\supermemo\systems\zenmode\elements\4\50.HTM
. This tells us where the HTML is stored on the disk. Since each Element is just an HTML, you can use different tools to manipulate the HTML content after identifying its file’s location.
How I Use It
My workflow mainly consists of two parts: first use an AHK script to get a branch’s Elements, then feed the list to Python for further processing. For example, I don’t like the added blue colon after splitting a Topic (see gif below).
I use the following AHK script to get all the Elements' locations, then I copy the string to the python script for find and replace execution.
1. AHK script: F1_Get_Element_Locations.ahk
What this script does:
-
Prompting for the number of times to go down the Knowledge Tree (KT)
-
Traversing the top Elements in KT to copy its HTML location
-
Save the raw string to Clipboard
To use it, with the starting Element in focus, press F1 (or any trigger):
The following is the end result: a comma-separated Python raw string:
|
|
2. Python script: batch find and replace with regex
traverse_html_regex_replace_and_replace.py
Explanation
|
|
For example, “C:\Users\user\Downloads\SuperMemo_Elements_Backup\”
|
|
These are regex. For more about regex, please see Regular Expressions: Regexes in Python
|
|
This is the list containing all the Elements' locations. Ideally it should be the result from the AHK script above.
|
|
Uncomment (remove the initial #) so that the script will also run the regex for removing the blue colon that are auto-generated from splitting a Topic.
For more info about what this script does, please read the docstring in the file.
PS: I’m aware that SuperMemo has built-in functions for find and replace, but it doesn’t support regex. For more sophiscated text manipulation, regex is a better tool.
PS II: I’m also aware that for proper HTML manipulation, one should use BeautifulSoup, but this script works good enough for me for now.
Conclusion
The takeaway is really about pressing ctrl+c
to copy the meta-data of the current Element.
This is just my particular workflow for handling and modifying the HTML. After getting the HTML locations, you can use any tools to manipulate the HTML: shell script, Python, JavaScript, etc. I use Python for its clean syntax and quick prototyping.